You need to have few simple steps to do this. You can just add a header to say that the page should output in excel format. But it is not effective since it will corrupt most of the time when the size is growing over 100kb. This way it allows you to output itas Binary. The other difference is this way you can output only the control you need. Not the whole page.
Step 1: Just copy paste the following method in to your page. This is just to allow the rendering engine to identify your control as a valid control. Dont do any change to this.
public override void VerifyRenderingInServerForm(Control control)
{
// Confirms that an HtmlForm control is rendered for the
// specified ASP.NET server control at run time.
}
Step 2: Add the code to your export button click event. Keep in mind to activate "ViewState" for the control. Note that "ReportTable" is the control I'm using.
protected void Export_Click(object sender, EventArgs e)
{
try
{
Response.Clear();
Response.ContentType = "application/vnd.ms-excel";
Response.Charset = "";
this.EnableViewState = false;
Response.AddHeader("content-disposition", "attachment;filename=Report.xls");
Response.Charset = "";
Response.ContentType = "application/vnd.xls";
System.IO.StringWriter stringWrite = new System.IO.StringWriter();
System.Web.UI.HtmlTextWriter htmlWrite = new HtmlTextWriter(stringWrite);
//GridView1.RenderControl(htmlWrite);
ReportTable.RenderControl(htmlWrite);
Response.Write(stringWrite.ToString());
Response.End();
}
catch (System.Threading.ThreadAbortException ev)
{
// Do nothing - This is comming from "Response.End();"
}
catch (Exception ex)
{
// Log any error message
}
}
Tuesday, January 29, 2008
Friday, December 7, 2007
Query full text table with RANKING
--Full text search query
select * from <FullTexed table name> O, CONTAINSTABLE(<FullTexed table name>, <FullTexed table column of your table>, '<search word>') AS K
WHERE K.[KEY] = O.<primary key of the table>
ORDER BY K.RANK DESC
Example:
select * from organisation O, CONTAINSTABLE(Organisation, Description, 'internet OR intranet') AS K
WHERE K.[KEY] = O.OrganisationID
ORDER BY K.RANK DESC
select * from <FullTexed table name> O, CONTAINSTABLE(<FullTexed table name>, <FullTexed table column of your table>, '<search word>') AS K
WHERE K.[KEY] = O.<primary key of the table>
ORDER BY K.RANK DESC
Example:
select * from organisation O, CONTAINSTABLE(Organisation, Description, 'internet OR intranet') AS K
WHERE K.[KEY] = O.OrganisationID
ORDER BY K.RANK DESC
Get DataBase info from queries
Get all SP names in a database
Simply run the below query under your database.
--Get all SP list of a DB
select name from sysobjects where type='p' order by name asc
Gt all Table of a DB
SELECT [A].* FROM [sysobjects] AS A where [Xtype] = 'U' order by [A].[name]
Gt all column names of a table
SELECT [syscolumns].* FROM [syscolumns], [sysobjects], [systypes] where [syscolumns].[ID] = [sysobjects].[ID] and [syscolumns].[xtype]=[systypes].[xtype] and [syscolumns].[id] = '<Table ID got from above query>' order by colid
Simply run the below query under your database.
--Get all SP list of a DB
select name from sysobjects where type='p' order by name asc
Gt all Table of a DB
SELECT [A].* FROM [sysobjects] AS A where [Xtype] = 'U' order by [A].[name]
Gt all column names of a table
SELECT [syscolumns].* FROM [syscolumns], [sysobjects], [systypes] where [syscolumns].[ID] = [sysobjects].[ID] and [syscolumns].[xtype]=[systypes].[xtype] and [syscolumns].[id] = '<Table ID got from above query>' order by colid
Monday, September 24, 2007
Wednesday, August 15, 2007
Mobile emulators in Visual Studio
This is how you can configure mobile device emulators in visual studio 2005.
1. install Microsoft "virtual pc" and "active sync"
2. open active sync and change dropdown to "DMA" and press ok
3. open device manager from .net IDE>> tools>> device emulator manager
4. right click the one you need to connect
5. select "connect". this will open the device interface
6. now right click the same item from the device manager
7. select "craddle". this will connnet your PC to the device.
now use the browser of the device to connect to your PC IIS. you need to use the MACHINE NAME as the sever name. (IP or LOCALHOST wont work) This can be used to develop and test windows and web applications for mobile devices.
1. install Microsoft "virtual pc" and "active sync"
2. open active sync and change dropdown to "DMA" and press ok
3. open device manager from .net IDE>> tools>> device emulator manager
4. right click the one you need to connect
5. select "connect". this will open the device interface
6. now right click the same item from the device manager
7. select "craddle". this will connnet your PC to the device.
now use the browser of the device to connect to your PC IIS. you need to use the MACHINE NAME as the sever name. (IP or LOCALHOST wont work) This can be used to develop and test windows and web applications for mobile devices.
Wednesday, August 1, 2007
Sql Server - BCP (Bulk copy program)
Bulk copy program is a very useful tool to be used when populating bulk data to the DB when load testing though it is not the intended usage of it. It created to set transfer bulk copies from server to any other in case of a batch transfer.
The simple command template is:
bcp <Db name>.dbo.<table name> in <file name> -c -t, -S<db server name> -U<user name> -P<password>
Exsample:
bcp MyDB.dbo.User out c:\users.txt -c -t, -S192.168.100.01 -Ume -Ptest
When you use "out", it will output table data to the specified file. By simply changing "out" to "in", you can easily input data from a text file you already have.
The above is for using sql server authentication to login to the server. If you need to use windows authentication, you need to add "-T" switch also. There are lot of other switches you can use with this command.
The simple command template is:
bcp <Db name>.dbo.<table name> in <file name> -c -t, -S<db server name> -U<user name> -P<password>
Exsample:
bcp MyDB.dbo.User out c:\users.txt -c -t, -S192.168.100.01 -Ume -Ptest
When you use "out", it will output table data to the specified file. By simply changing "out" to "in", you can easily input data from a text file you already have.
The above is for using sql server authentication to login to the server. If you need to use windows authentication, you need to add "-T" switch also. There are lot of other switches you can use with this command.
Monday, July 30, 2007
Show child object properties in GridView
Here we have a simple trick.
I'll introduce my objects first.
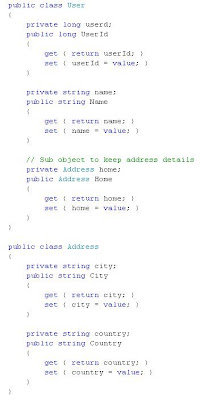
Nothing to worry about. If I show you the XML view of the User[], it should looks like below.
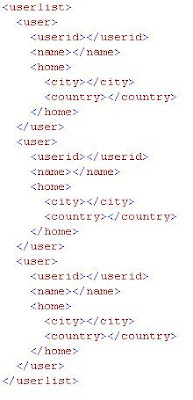
Now we can assign this to a GridView.
Code:
GridView1.DataSource = UserList;
GridView1.DataBind();
Design:
Dont forget to import the objects namespaces in the page header in the HTML view.

........
........
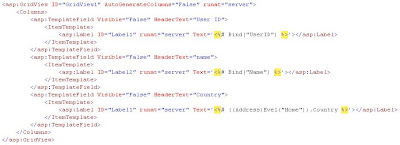
Explanation:
I'm using
to show the child object property values in to the grid.

What we are doing here is to match the Parent object property name Home to access the child object. Actually it is a object of Address type. So that I can cast the object to Address type and access the child property Country.
I'll introduce my objects first.
Nothing to worry about. If I show you the XML view of the User[], it should looks like below.
Now we can assign this to a GridView.
Code:
GridView1.DataSource = UserList;
GridView1.DataBind();
Design:
Dont forget to import the objects namespaces in the page header in the HTML view.
........
........
Explanation:
I'm using
What we are doing here is to match the Parent object property name Home to access the child object. Actually it is a object of Address type. So that I can cast the object to Address type and access the child property Country.
Subscribe to:
Posts (Atom)